How to structure Programming Projects?
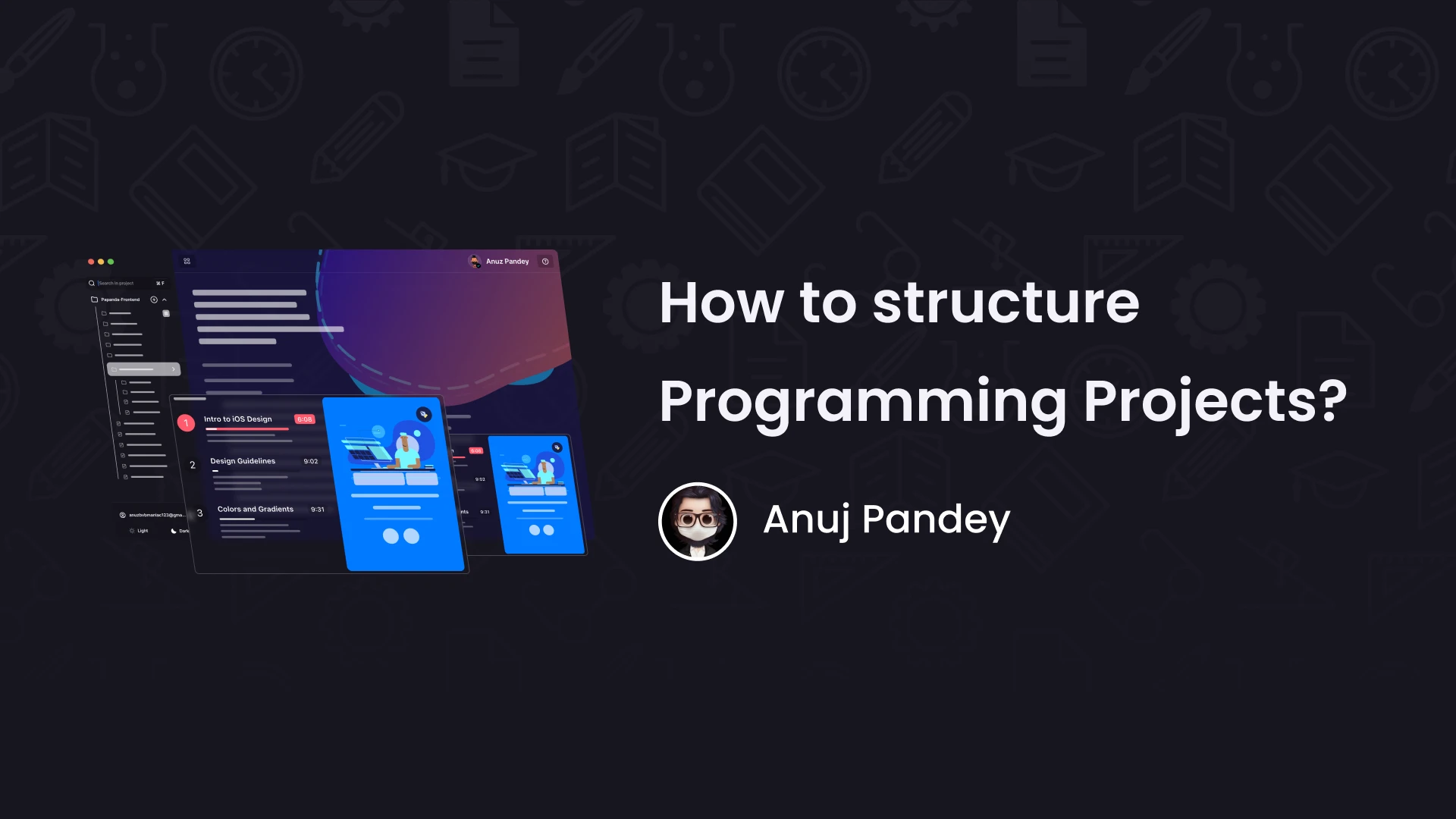
A friend of mine asked me what is the best way to set up a programming project from the start. This article will help you to speed up your workflow by making you a way more efficient programmer. The tools and techniques described in this article will help you maintain structure and organize your programming project.
Intro
So, have you ever had the experience of coming up with an idea for a project that you want to build and then being so excited about it, you want to start working on it right away? After a few hours or days, you find that the project you thought would be two files and a few lines of code has grown into a project with fifteen files and hundreds of lines of code. You know a lot of the files includes the code that's no longer in use, but you're not sure which ones, so you don't want to remove any of them because you don't want to damage the entire code base, and you also know there's a bug somewhere in this massive jumble of code that needs to be fixed.
This has happened to me in the past and a buddy of mine was in a similar scenario and asked, "What is the best approach to set up a programming project so that the organization maintains its structure from the start?" So that's what I came up with, and I though I'd assist you in answering it in this article.
The most efficient method of structuring the Programming Project
- How to build up your project's folder structure and the best practices to follow.
- How to utilize Github Workflow for project management and version control.
- Selecting the Most Appropriate Text Editor for the Job
- Creating flows for developing good code and keeping your codebase up to date.
The tools and strategies presented in this article will help you keep your programming project organized and structured, as well as assist you speed up your workflow by making you a more efficient coder.
So let's get this started. Based on my findings, the followings are the workflow that I recommend.
Create the project's primary folder.
Make a folder with the project's name. In order to have full control over your project, create a Git repository within the project folder. It is critical that you do it right away.
After that, we'll need to organize the folder within this project folder. To begin, we'll make a readme file in the project folder. A readme file is a file that can be utilized in a variety of ways. This is mostly used as a file to lay down the project's basic information so that others may read the readme file and understand what your project is all about. It's also a best place to write some basic instructions for getting the project up and running. In some ways, it's like a cheat sheet for installation.
A simple readme structure could include the "Title of the Project," a brief explanation of the project, and an Installation Guide, which lists all of the steps required to get the project up and running. I recommend creating a separate Installation Guide file for larger projects where we put out all of the directions for getting everything installed and set up. However, you can put all of this in the readme file in general. For the more OCD people out there, the separate Installation file is essentially simply an added degree of structure.
After that, we can make our first commit, which will be a conventional "Added a Readme File" commit.
The next thing we'll do is create folders or directories, or whatever you want to call them. And now this is where I propose doing a quick Google search for the framework or languages that you'll be using, followed by the gitignore file that those framework uses.
I would propose making two folders inside main directory:
- tests/
- src/
The src/source folder is essentially where your source code files will be saved. Some people may think that having this folder is redundant, but I like to use it since it gives a little bit of organization to our code base and also allows me to discover what we need within our codebase much more quickly. If you're working on a larger project that will require a lot of files, I strongly advise you to create sub-folders within the /src folder. And the folders here will depend on a lot on the needs for your specific project. The MVC protocol, which stands for Model View Controller, is the most common project structure that we commonly use. This is a standard format for most of the web apps, mobile apps, and anything else that has a user interface. This is also suitable for back-end development. So this will be the basic folder structure for our project.
We may now push a new commit to our master branch indicating that we've added some folders to our project.
So that's the gist of what we'll need on our machine locally. The Github repository is up and running, and we've organized our folders in a way that will help us keep organized as we write our code.
The next thing I recommend is creating a project board for our repository on github.com. This is something I believe is such an amazing tool since it will help us keep track of what we should and shouldn't be doing, plus with the Github project board, we can do a ton of really cool things that integrate smoothly into your repository. Github Project Board can be used to track our progress and manage projects.
We can establish a Kanban-style task management structure in which we create issues for any item that we believe needs to be completed right now, with an issue being nothing more than a TO-DO item. It could be thought of as a specific functionality, such as setting up a flutter project, creating a login screen, configuring login logic, and so on.
Now that we have these, we can start working on each task independently, which will help us retain that structure when writing our actual code, which is crucial since we want to keep our scope for the code we write as small as possible. This means we should only write or work on one task at a time, rather than multitasking. When we write code for our own projects, we don't usually have this kind of structure, so we end up working on ten different features at once, which leads to a lot of messy code and errors down the road.
I recommend making it a habit to open this page every time we sit down to code and update it on a regular basis. This leads in a large number of smaller tasks that are much more manageable than sitting down and thinking about the big project we have in front of us, where we have no idea where to begin and start from.
Instead of having one to-do on our list that says " Clean Apartment," which can feel like a herculean task, we can have lots of tiny to-dos like " Clean your desk." " Put the trash out." " Fold your clothing." and so forth. Each task becomes less overwhelming and more manageable as a result. I strongly advise you to do this even on the smaller projects you work on, where it may seem like overkill at first, but you should get into the habit of performing these things on autopilot.
We may then return to our local PC and create a new git branch called "release." If you're working on a small project or if you're the only one working on it, you can skip this step. I recommend that you do so, so that when you work on a huge project with other people, you are used to working in the right and proper way.
Following that, we may build a new branch, which will be the "feature" branch. This section is dedicated to the features we'll be developing. As an example, the name of this branch could be "flutter-project." We'll be writing your code in the "Feature" branch. This is also a great technique to ensure that we stay focused on one task at a time and don't go off on a track and keep writing hundreds of lines of code for three distinct features at the same time. Impatience and a lack of structure cause a lot of difficulties in programming, which leads us to the following point, which is about optimizing the way we create our code.
"Use the every other day system", which implies that every other day you sit down to code with the sole objective of correcting your code, which does not necessarily mean fixing your bugs, but rather reworking and rewriting your functioning code to make it more efficient. We all know that after six hours of attempting to fix an issue, we get a little impatient and start commenting out code that doesn't work and maybe even rewrite entire functions simply to get things work, leaving the old functions hanging around doing nothing. We also create temporary variables that we never erase, and all of this adds up to unnecessary print statements being used as a sort of poop man's debugging system at the last minute or as a last option. So, spending time removing these things and simplifying the code we create goes a long way, so I recommend that you sit down every other day or once a week and devote the full day to rewrite your clunky code. This can be quite beneficial in terms of maintaining the project's readability over time. The added benefit is that you will learn how to develop more efficient code.
The second thing I encourage is following naming conventions. So, instead of calling your variable test 1 or hello1, call your functions, function or calculate, spend a few seconds coming up with a descriptive name rather than a snappy name which will save you a lot of time.
Finally, I would recommend that you select the appropriate text editor for the job. I presently use sublime text for minor projects where I know it will just be a quick little script that will only require one file, and Intellij Products for larger projects.
Okay, That's all I have to say about it. I hope you enjoyed reading it and will like, share, and comment on it. Thank you for taking the time to read this. Stay Healthy, Stay Safe.