Laravel Blade Directives: Authorization
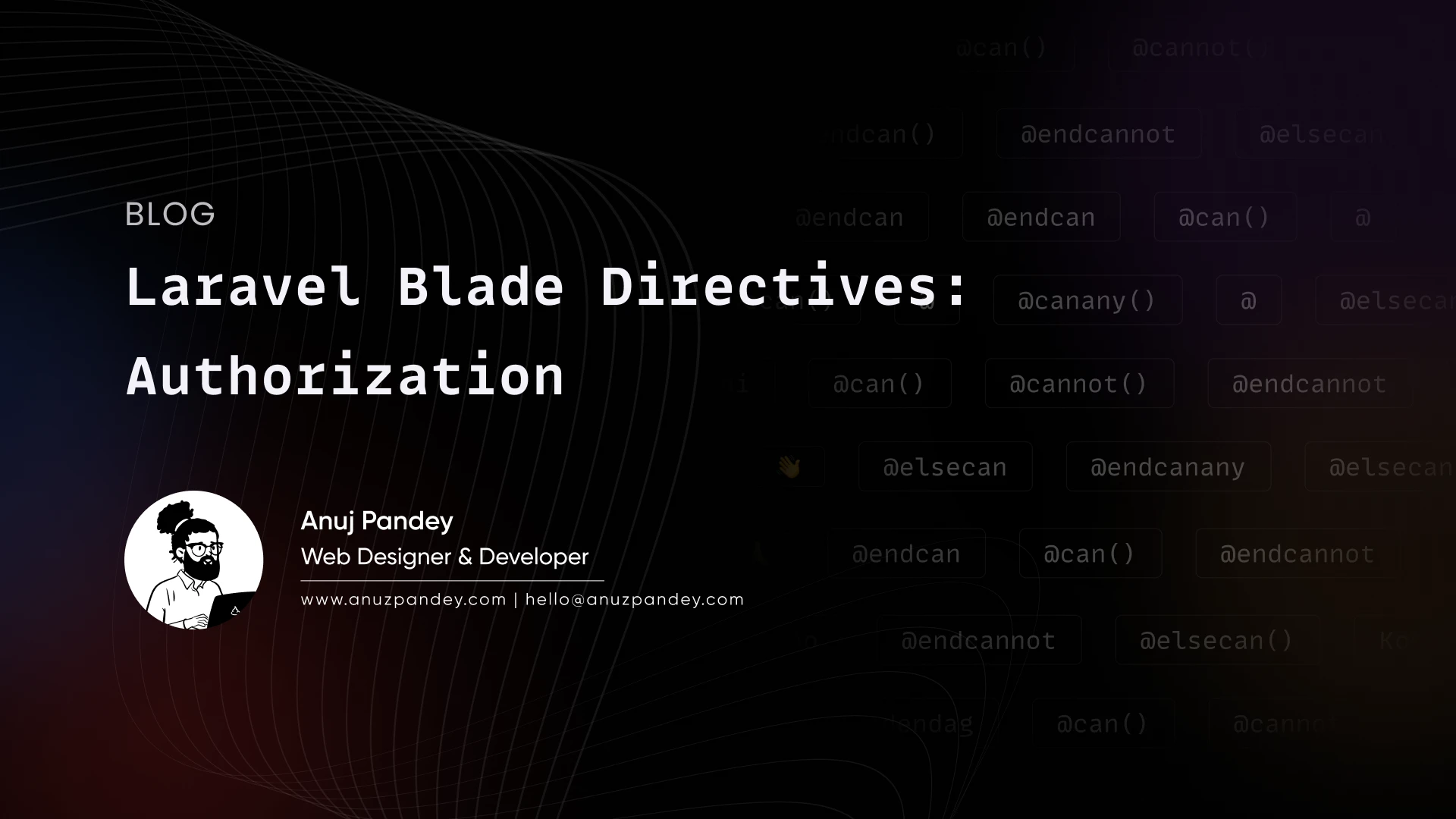
In Laravel, the Blade templating system provides several directives for authorization. These directives allow you to conditionally display content based on the user's permissions. The directives @can, @cannot, @canany, @elsecan, @elsecannot, @elsecanany, @endcan, @endcannot, and @endcanany are used to manage and handle authorization within your Blade templates. They're typically used to organize authorization logic and control the access to the user on certain scenario. These are typically done with Gate and/or via Policy classes in Laravel.
For more detailed information about authorization in Laravel, you can refer to the official Laravel documentation. The specific section dedicated to authorization can be found here. This resource will provide you with a deeper understanding of how Laravel handles authorization, and how you can effectively use it in your applications.
Now, let's dive into different Blade directives for authorization checks that Laravel provides us out of the box.
@can
The @can directive is used to determine if a user is authorized to perform a given action. It's often used in the context of a model, to check if a user has a certain ability or permission related to that model. If the user is authorized, the code within the @can and @endcan directives will be executed.
Sure, here is an example of how you might use the @can directive in a Blade file:
In this example, edit is the ability we are checking for, and $article is the relevant model instance. This will only render the "Edit Article" link if the current user has the 'edit' ability for the given $article.
@elsecan
The @elsecan directive is used for authorization within an @can statement which provides an alternative block of code that will be executed if the previous @can condition was not met. Here is an example of how you might use the @elsecan directive in a Blade file:
In this example, if the current user does not have the 'edit' ability for the given $article, it then checks if the user has the 'view' ability. If the user has the 'view' ability, the "View Article" link will be rendered.
@cannot
The @cannot directive is the exact opposite of the @can directive. It determines if a user is not authorized to perform a given action. If the user is not authorized, the code within the @cannot and @endcannot directives will be executed.
Here is an example of how you might use the @cannot directive in a Blade file:
In this example, edit is the ability we are checking for, and $article is the relevant model instance. This will render the message "You do not have permission to edit this article." if the current user does not have the 'edit' ability for the given $article.
@elsecannot
The @elsecannot directive provides an alternative block of code that will be executed if the previous @cannot condition was not met. Here is an example of how you might use the @elsecannot directive in a Blade file:
In this example, if the current user does not have the 'edit' ability for the $article, it then checks if the user has the 'view' ability. If the user does not have the 'view' ability, the message "You do not have permission to view this article." will be rendered. This directive helps in defining alternate conditions and actions in the case when a user lacks certain permissions.
@canany
The @canany directive is used to check if a user has any of the given abilities. It accepts an array of abilities and a model as arguments. If the user has any of the provided abilities, the code within the @canany and @endcanany directives will be executed.
Here is an example of how you might use the @canany directive in a Blade file:
In this example, edit and create are the abilities we are checking for, and $article is the relevant model instance. This will render the "Edit Article" and "Create article" links if the current user has either the 'edit' or 'create' ability for the given $article.
@elsecanany
The @elsecanany directive is used within an @canany statement which provides an alternative block of code that will be executed if the previous @canany condition was not met. Here is an example of how you might use the @elsecanany directive in a Blade file:
In this example, if the current user neither has the 'edit' nor 'create' ability for the given $article, it then checks if the user has the 'view' ability. If the user has the 'view' ability, the "View Article" link will be rendered.
Conclusion
In this article, we've explored different Blade directives for authorization checks in Laravel. These directives provide a convenient way to manage and handle authorization within your Blade templates. By using these directives, you can conditionally display content based on the user's permissions, and organize authorization logic in your application.
If you have any questions or need further clarification on any of the topics discussed in this article, feel free to reach out to me. I'll be happy to help you out.
Keep coding, keep exploring, and keep inspiring. 🐼