Laravel Blade Directives: Conditionals
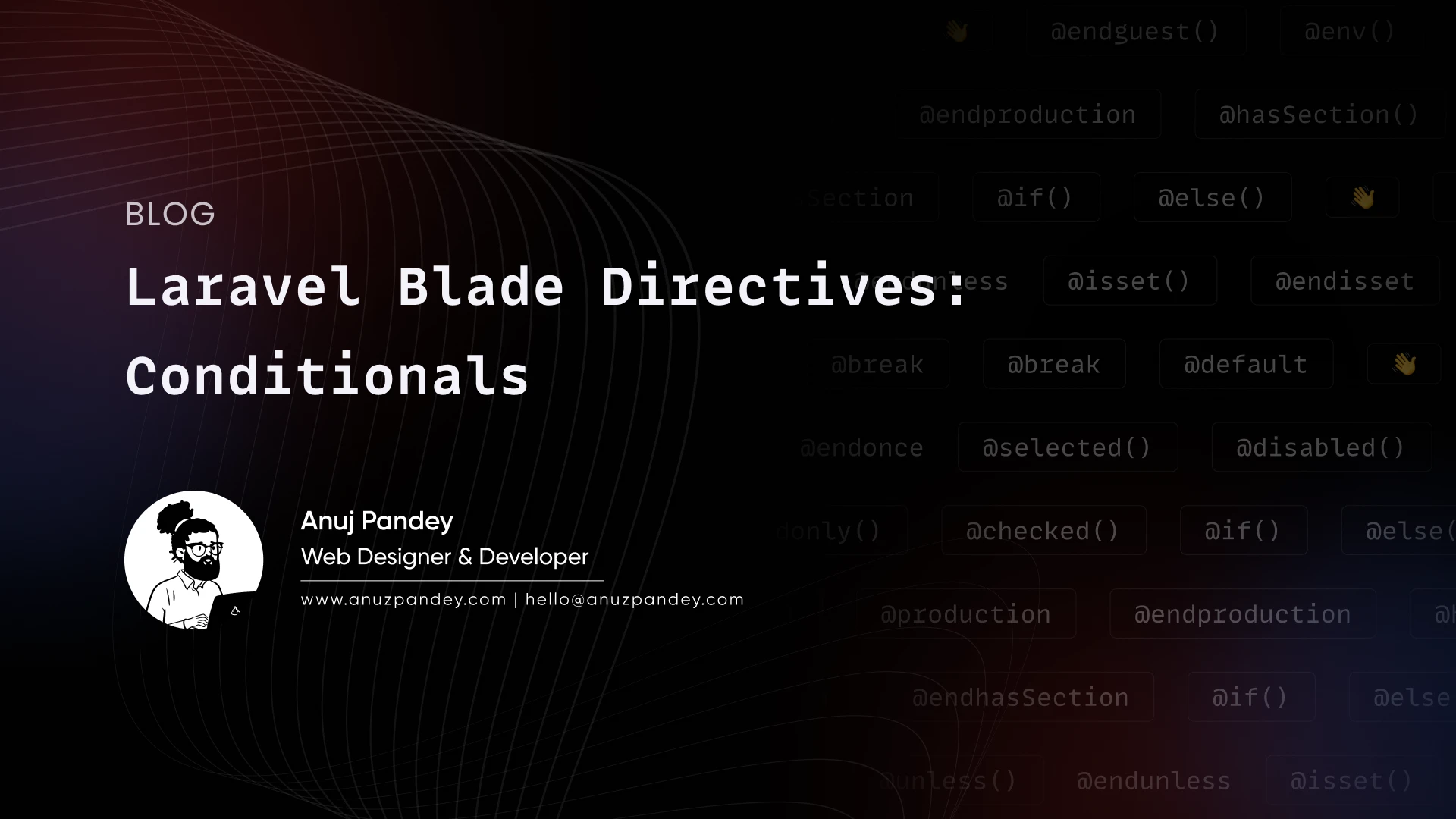
Auth @auth(), @elseauth(), @endauth
These directives are used to control the content visibility based on the user authentication status.
- auth() - Checks if the user is currently authenticated (logged in), and if the user is logged in the content within this block is displayed in the view. This directive can also take an input which must be a valid guard name.
- @elseauth - This directive is used in conjunction with @auth. If the user is not authenticated (i.e., not logged in), the content inside this directive will be displayed. Same as auth , this directive can take a guard name as input and control the content visibility.
- @endauth - This directive marks the end of the @auth directive block.
As shown in the example, you can also use @else directive to have more control of the content on the view.
Guest @guest(), @elseguest(), @endguest
The @guest directive is the exact opposite of @auth directive. The @guest, @elseguest, and @endguest directives are used to conditionally display content based on whether the user is a guest (not authenticated).
- @guest: This directive checks if the user is a guest (not authenticated). If the user is a guest, the content inside this directive will be displayed.
- @elseguest: This directive is used in conjunction with @guest. If the user is not a guest (i.e., authenticated), the content inside this directive will be displayed.
- @endguest: This directive marks the end of the @guest directive block. guest and @elseguest directives can also take an input which must be a valid guard name.
Env @env(), @endenv
The @env and @endenv directives are used for conditional logic based on the application environment in Laravel Blade templates.
- @env - This directive starts a conditional block. It can take arguments in different formats:
- @env('production') - Checks if the current environment matches the specified string.
- @env(['local', 'staging']) - Checks if the environment matches any of the listed strings.
- @endenv - This marks the end of the conditional block started by @env.
Production - @production, @endproduction
The @production and @endproduction directives in Blade templates are a specific case of the more general @env and @endenv directives. They provide a shorthand way to conditionally include contents only when the application is running in the production environment.
- @production - This acts as a shortcut for @env('production'). It starts a conditional block that executes only if the current environment is set to "production" (as defined in your .env file).
- @endproduction - This marks the end of the conditional block started by @production.
Has Section - @hasSection()
The code snippet @hasSection() is used to conditionally render content based on the existence of a specific section.
- @hasSection('header'): This directive checks if a Blade section named "header" has been defined within the current template or a parent template.
- Sections are reusable blocks of code that can be defined using @section and @endsection directives.
- @endif: This directive marks the end of the conditional block started by @hasSection.
The code essentially creates an if-else statement:
- If a section named "header" exists (i.e., has been defined using @section('header') ... @endsection), the content between @hasSection('header') and @endif will be rendered.
- If no "header" section is found, the content within the block is skipped. This way, you can create a consistent layout across your views while allowing for customization of specific sections like the header.
Section Missing - @sectionmissing()
The code snippet @sectionmissing() is used to conditionally render content specifically when a section is not defined. Here's a breakdown:
- @sectionmissing('header'): This directive checks if a Blade section named "header" is missing from the current template and its parent templates.
- @endif: This directive marks the end of the conditional block started by @sectionmissing. Similar to @hasSection, this code creates an if-else statement, but with the opposite condition. This directive is helpful for providing default content or handling situations where a specific section might not be defined in all views.
This way, you can ensure a default header is displayed even if the view doesn't define it explicitly.
If-Else Statements
@if(), @elseIf(), @else, @endIf represents a conditional statement structure used in Laravel Blade templates. It's functionally equivalent to standard PHP's if-else-elseif statements but with Blade syntax.
- @if(): This initiates the conditional block. The parentheses contain an expression that needs to be evaluated as true or false.
- If the expression evaluates to true, the code within the @if block will be executed.
- @elseIf(): This is an optional block used for additional conditional checks. It allows you to define multiple conditions to be evaluated sequentially.
- The parentheses hold another expression. The code within this block will only be executed if the condition in the previous @if or any preceding @elseIf blocks were false.
- @else: This is also an optional block that serves as a catch-all.
- The code within @else will be executed only if none of the conditions in the preceding @if or @elseIf blocks were true.
- @endIf: This marks the end of the entire conditional statement structure.
This is a basic example, and you can chain multiple @elseIf blocks for more complex conditional logic within your Blade templates.
Unless - @unless(), @endUnless
The @unless and @endUnless directives provide a concise way to write conditional statements based on the negation of a boolean expression. It's essentially a shortcut for a common use case of @if.
- @unless(): This initiates the conditional block. The parentheses contain an expression that needs to be evaluated as true or false.
- Unlike @if, the code within the @unless block will be executed only if the expression evaluates to false.
- @endUnless: This marks the end of the conditional block started by @unless.
@unless improves code readability by expressing the condition in a more natural way when you want to execute code if something is not true. It reduces verbosity compared to writing @if (! $expression) ... @endif.
Using @unless makes the intent clearer: we only want to show the link if the user is not logged in. Remember, the logic within @unless executes only when the condition evaluates to false. It's a convenient way to write conditional statements focusing on the negative case.
Isset - @isset(), @endIsset
The @isset and @endIsset directives are used to check if a variable is set and is not null. It offers a concise way to handle situations where you need to avoid errors caused by accessing properties or methods on variables that might not exist.
- @isset helps prevent errors by ensuring a variable exists and has a value before attempting to use it. This improves code safety and avoids potential undefined variable errors.
- It offers a more concise syntax compared to using isset($variable) directly within an @if statement.
@isset can handle multiple variables in a single check by separating them with commas within the parentheses. The condition is true only if all listed variables are set and not null. For more complex checks involving multiple conditions, you can combine @isset with other conditional directives like @if or @else.
Switch Statements
The @switch directive in Laravel Blade templates along with @case , @break and @default allows for conditional code execution based on the value of an expression. It's a Blade-specific syntax that mimics switch statements.
Here's a breakdown of the directives:
- @switch($expression): This initiates the switch statement. The parentheses contain an expression that will be evaluated, and the result will be used for matching against the cases.
- @case($value): This defines a case block. The parentheses contain a specific value to be matched against the expression's result. You can have multiple @case blocks to handle different match conditions.
- @break: Used within a @case block to exit the switch statement immediately after a matching case is found. This prevents code from executing through subsequent @case blocks.
- @default: This is an optional block that serves as a catch-all. The code within @default will be executed if none of the conditions in the preceding @case blocks were a match.
- @endSwitch: This marks the end of the entire switch statement structure.
- @switch provides a more concise and readable way to write conditional logic compared to nested @if statements.
- The @break directive (optional within each @case block) is used to exit the switch statement after a matching case is found. This prevents code from falling through to subsequent cases.
- Similar to @unless, @switch can improve readability by focusing on the positive cases (matched values) with @case and handling the default case (no match) with @default.
Once - @once(), @endonce
The @once and @endonce directives in Laravel Blade templates offer a way to ensure a block of code is executed only once during the template rendering process. This is useful for avoiding duplicate content or logic execution, especially when dealing with loops or conditional inclusions.
- @once: This initiates the block where code should be executed only once.
- @endonce: This marks the end of the code block controlled by @once.
@once directive improves performance by avoiding unnecessary processing and enhances code maintainability by ensuring logic is executed only at the intended location.
@once works by storing a flag in the Blade template cache to track if the block has already been executed. It's particularly helpful for including JavaScript or CSS files that should be loaded only once within a template. While @once is convenient, for more complex scenarios involving conditional logic within the block, you might still need traditional @if or @switch statements for finer control over code execution.
Form Element Attributes
These directives in Laravel Blade templates are all related to handling HTML form element attributes. They provide a concise way to conditionally apply these attributes based on logic within your Blade views.
Here's a breakdown of each directive:
Selected - @selected()
Checks if the value of the option element matches the provided value as an argument within parentheses. If there's a match, the selected attribute is added to the element, making it the pre-selected option when the page loads.
Checked - @checked()
Checks if the provided value (as an argument within parentheses) matches the element's value. If there's a match, the checked attribute is added to the element, making it pre-selected when the page loads.
Required - @required()
Adds the required attribute to the element, if the condition or boolean expression passed as argument is true.
Disabled - @disabled()
Adds the disabled attribute to the element, if the condition or boolean expression passed as argument is true.
Readonly - @readonly()
Adds the readonly attribute to the element, allowing the user to see the value but preventing modifications, if the condition or boolean expression passed as argument is true.
These directives offer a clean way to manage form element attributes based on conditions within your Blade templates. They improve code readability and maintainability compared to manually adding or removing attributes based on logic.
Conclusion
Laravel Blade directives are a powerful tool that enhances the development experience. By leveraging these directives effectively, you can create well-structured, maintainable, and expressive templates that contribute to the overall quality and readability of your Laravel application.
If you have any questions or need further clarification on any of the topics discussed in this article, feel free to reach out to me. I'll be happy to help you out.
Keep coding, keep exploring, and keep inspiring. 🐼