Laravel Blade Directives: Loops
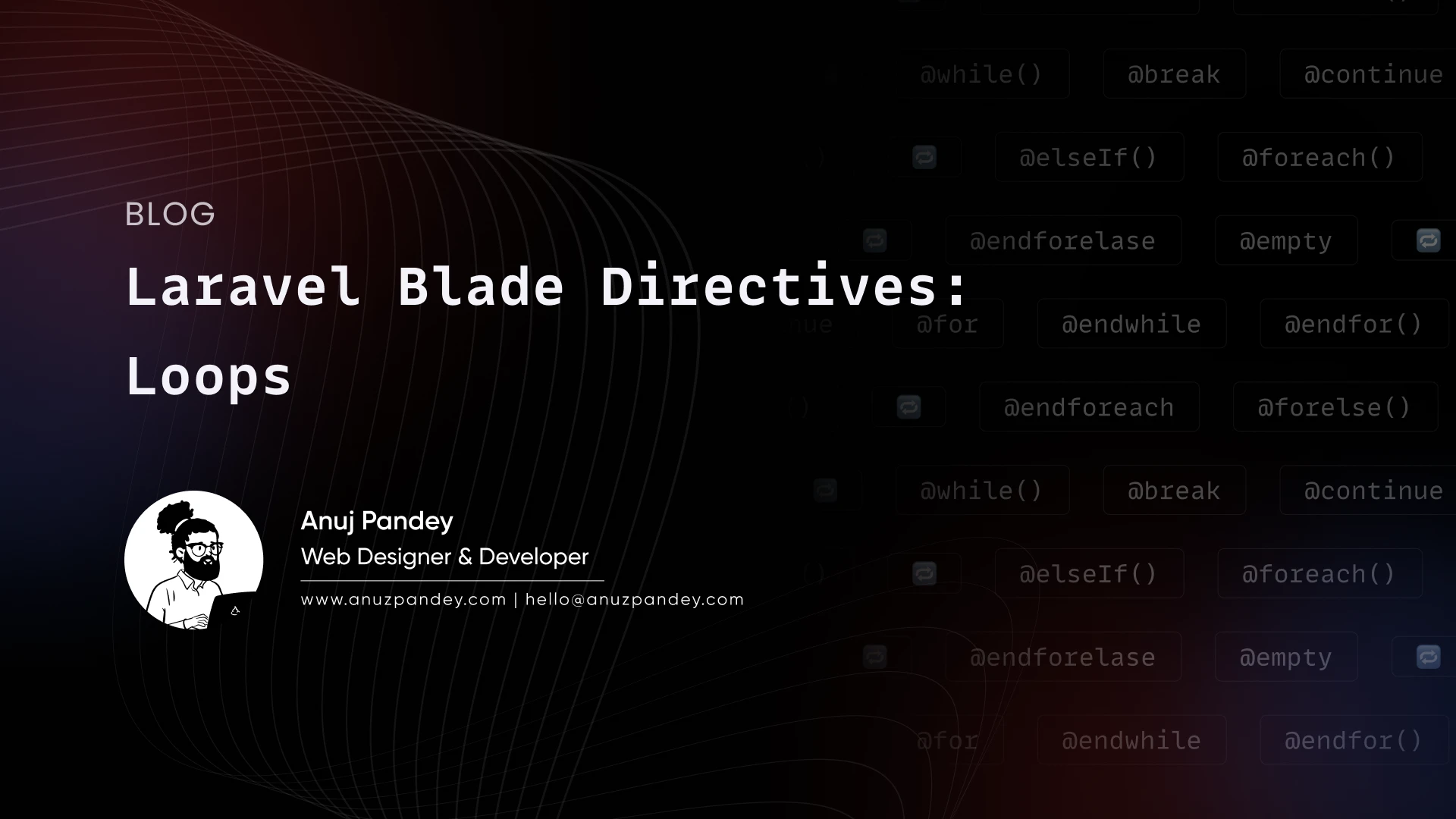
Laravel provides us some blade directives to work with loops. These directives allow you to iterate over arrays or objects, handle empty states, and control loop behavior. In this article, we will learn about the following Blade directives for loops.
Foreach - @foreach, @endforeach
- Used for iterating over arrays or objects in your Blade templates.
- Functionality:
- @foreach($collection as $keyVariable => $itemVariable):
- Initiates the loop.
- Takes two arguments:
- $collection: The name of the array or object to iterate over.
- $itemVariable: The variable name to hold the current item during each iteration.
- $keyVariable (Optional) : An optional variable name to hold the current key/index for each item.
- Loop Body: The code between @foreach and @endforeach gets executed for each item in the collection. You can access the current item's data using $itemVariable. You can access the current key/index (if provided) using $keyVariable.
- @endforeach: Marks the end of the @foreach loop.
- @foreach($collection as $keyVariable => $itemVariable):
This example iterates over a collection named $users. For each user, it displays their name and email within a "user" class div. You can see the $loop variable used in the @foreach() and @endforeach block. $loop is a special variable injected within the @foreach loop construct in Laravel Blade templates. It provides access to meta-information about the current iteration of the loop, allowing you to perform additional actions or customize behavior based on the loop's progress.
For more details, you can refer to the official Laravel documentation: Loop Variable or you can refer to our article: Laravel $loop Variable.
Forelse - @forelse, @empty, @endforelse
These directives provide a concise and efficient way to iterate through a collection of data in your Blade templates, handling both the case when there are items in the collection and the case when it's empty.
Explanation:
- This code iterates through a collection named $products.
- For each product, it displays its name, description, and a link to its details page.
- If $products is empty, it displays a message indicating no products were found.
For more details on $loop variable, you can refer to the official Laravel documentation: Loop Variable or you can refer to our article: Laravel $loop Variable.
For - @for, @endfor
- Used for traditional for loops based on a counter variable and specific conditions.
- Functionality:
- @for($counter = 0; $counter < 10; $counter++):
- Initiates the loop with a starting value, condition, and increment/decrement expression.
- Loop Body: Similar to @foreach, the code between @for and @endfor executes for each iteration based on the loop condition.
- @endfor: Marks the end of the @for loop.
- @for($counter = 0; $counter < 10; $counter++):
This example uses a for loop with a counter variable $i that starts at 1, runs as long as $i is less than or equal to 5, and increments $i by 1 in each iteration. This code creates five checkbox inputs with values 1 to 5.
While - @while, @endwhile
- Used for loops that continue executing as long as a specific condition remains true.
- Functionality:
- @while($condition):
- Initiates the loop based on a condition.
- Loop Body: Similar to @foreach, the code between @while and @endwhile executes repeatedly as long as the $condition evaluates to true.
- @endwhile: Marks the end of the @while loop.
- @while($condition):
This example keeps looping as long as the count($errors) is greater than 0 (meaning there are validation errors). Within the loop, it iterates through all error messages from the $errors object and displays them in a list.
Continue - @continue
- Used to skip the remaining code within the current loop iteration and move on to the next iteration.
This example iterates through orders. If an order's status is "cancelled", it skips the rest of the code within the current iteration using @continue and moves on to the next order.
Break - @break
Used to immediately terminate the current loop iteration, exiting the loop completely.
This example iterates through products. If a product's stock is 0 (out of stock), it displays an "Out of Stock" message and uses @break to immediately exit the loop, stopping further product display.
Conclusion
In this article, we learned about Laravel Blade directives for loops. We covered @foreach, @forelse, @empty, @for, @while, @continue, and @break. These directives provide a powerful and concise way to work with loops in your Blade templates, making it easier to iterate through collections, handle empty states, and control loop behavior.
If you have any questions or need further clarification on any of the topics discussed in this article, feel free to reach out to me. I'll be happy to help you out.
Keep coding, keep exploring, and keep inspiring. 🐼